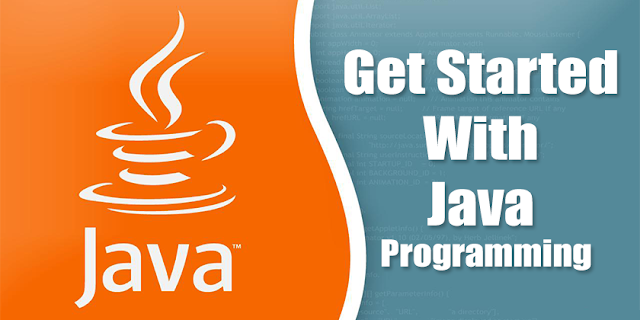
The first question, what is this Java stuff anyway… well Java is an “Object Oriented Programming”(OOP) language. We will know about OOP in the next part, first of all we must know what is a programming language… It’s actually giving the computer instructions to carry out specific job, or “teaching the computer several ways to solve problems”. If you don’t have any programming experience, then let me tell you to write any program think how do you yourself will solve it in real world, then translate your thoughts in the programming language. So let’s start with Java…
History and Usage
The team which created Java was under James Gosling, and they worked for Sun Microsystems to create a platform-independent programming language. The first implementation was done in the fall of 1992, then it was called ‘Oak’ but the name was later changed to ‘Java’ due to some legal snags. The first public announcement of Java was done in 1995.Since then Java turned out to be a global hit, it was a revolution in internet for its applets. Major companies like Google and Amazon extensively uses Java. Also java produced maximum number of jobs in 2013.
How Java works
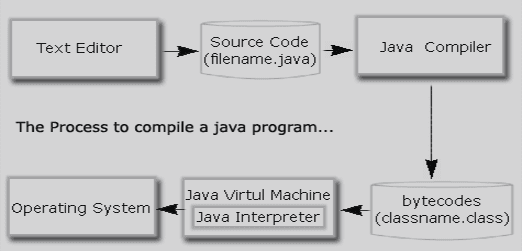
The legend of Java is in “Byte Code”. When we write a Java program we store it in a file with .Java extension, after compiling we get a file with .class extension. This is the portable file which holds the byte code, which can be taken to any computer with any platform and be run. The byte code is interpreted with JVM (Java Virtual Machine), which converts all the Java code to machine. Thus, Java is both compiled and interpreted language.
SOURCE CODE»javac(JAVA COMPILER)»BYTE CODE»JVM»MACHINE CODE.
Concepts Of OOP
If you are already versed with the concepts of OOP or done any other OOP language such as C++, you may skip this part.The main concept of OOP is PIE, or Polymorphism Inheritance Encapsulation. We will now deal with these heavy looking terms.

Polymorphism
Lets take the word ‘cell’ we use it to define the structural and functional part of living things or a mobile phone or a jail room or intersection of rows and columns in excel. You can see that a same word is used to define many things, this is the concept of polymorphism. We can do the same in java, the practical part of this comes in the later tutorials.
Inheritance
A son has many characteristics of his parents and this is only the general idea of inheritance, the same applies to programming, its carrying common attributes or behaviours from one entity to another.Encapsulation
Its packing several attributes or entities in an single entity, for example we have several words- Plant, Animal, digestion, stomata, stomach, transpiration, we can see the are jumbled words with plant and animal organs and processes. This is what happens in Procedure Oriented Programming, but inOOP we don’t let them float around but we pack them. Animal{digestion(stomach)} Plant{transpiration(stomata)}. In programming terms Animal and Plant are classes, digestion and transpiration functions and stomach and stomata data members. We will discuss these terms later on in detail.
Java Classes, Functions and Objects
Before we start coding we must know what these are. Well class is a collection of data, functions, etc. actually it is the encapsulating entity. It is also called the object factory, a collection of classes is known as package.Objects contains all the characteristics of a class, any function or data member of the class it belongs to can be accessed through it
(Though it depends on the visibility modes, about which we will discuss in later tutorials).
Functions are the code blocks in which the work is done, we write the code to be executed in functions.
We may take Car as a class, BMW and Ferrari can be considered its objects and driving its function.
The First Java Program
Let’s now start up with coding (finally..!!). Here is a very very simple Java program, have a look:
class
Techmartz {
public
static
void
main(String h[]){
System.out.print(
"Hello world from Techmartz"
);
}
}
Output:
Now let’s have a post-mortem of the program. We begin with the class keyword, it declares a class called CodeNirvana… Here’s the syntax- class<class name>.
We may give any class name but it is the convention to begin the class name with a capital letter and start every new word with a capital letter.Next we write a brace to begin defining the class in Java we start a code block with a { and end it with a }. The code block opened at last must be closed at first.
Then we write the word “public static void main(String h[])”, we will discuss these words in detail in our tutorial about functions. For now, you should know that these words are to define a function called “main” from which most of the Java programs starts to execute. Remember, when you code in general, then “main” method is must.
Then comes the important part of the System.out.print(); statement, this is the displaying statement of Java, whatever letters we want to write on the console we will write it in double quotes. System.out.print(); displays the content but dose not takes the cursor to the next line, whereas System.out.println(); displays the content and takes the cursor to the next line.
You may notice the “;” after the statement, the semicolon is used to terminate each statement, behold my friends!! This is the place where most programmers get their bugs.
Using Numbers and Variables
We will discuss about all variable types in the next tutorial, here we will use only the “int” type. First of all, what is a variable? Well, they are named memory spaces to store data while running the program.
Syntax to declare a variable: <data type> <variable name>;
Assign it a value: <variable name>=<value>;
lets join it..
<data type> <variable name>=<value>;
Example: int a=10;
Remember a variables value can be changed any time but the declaration has to be made only once.
We may declare multiple variables with same data type like this…
int a=0,b=1,c=9………z=7;
Every variable in Java has a data type, Here we use “int” it is to store an integer constant.
Few Operators with variables
Let’s use the basic mathematical operators now.
int a=10+5;now, a=15
int b=a*10;
now b=150;
Simple, isn't it.
System.out.print() with variables.
To display any variable we don’t need to give the double quotes, let’s take an example
int a=25;
System.out.print(a);
Outpur: 25.
We may do a calculation:
int a=5;
int b=10;
System.out.print(a+b);
Output: 15
We may display a variable’s value along with letters using the ‘+’ operator like this:
int a=100;
System.out.print(“Hundred=”+a);
Output: Hundred=100
But to do a calculation along with displaying a string(Group of letters) needs another bracket:
int a=5,b=10;
System.out.println(“Sum=”+a+b);
System.out.print(“Sum=”+(a+b));
Output:
Sum=510
Sum=15
Beware of this mistake:
int a=10;
System.out.println(a);
System.out.print(“a”);
Output:
10
a
Remember whatever we write in double quotes will be displayed as it is(Except the escape sequences…don’t worry, this all ill come in the third tutorial)
Running the Java program
Best IDE for Java Programming: Visit Here
First we have to write the code in any text editor like notepad or notepad++, then save it with the class name as the file name and with .Java extension. Now where to save it? It should be saved in the bin folder of the jdk’s folder in your Java root directory. The path in Windows XP using jdk1.7 update 45 is: C:\Program Files\Java\jdk1.7.0_45\bin
Or, you may change your “path” environment variable to the path of your bin folder like this in command prompt: set path= “C:\Program Files\Java\jdk1.7.0_45\bin”Next you must compile the program with javac command like this
Example:
javac “C:\Program Files\Java\jdk1.7.0_45\bin\Techmartz.java”
If there is any syntax error it will be displayed else a .class file will be created. Now to run it we will use the Java command after navigating to the directory where the .class file is.
java <class name>
Example:
java Techmartz
Your program will now run successfully…
Sign up here with your email
ConversionConversion EmoticonEmoticon